Today, We want to share with you Angular 6 PHP CRUD (Create, Read, Update, Delete) Operations.
In this post we will show you ANGULAR 6 PHP MySQLi CRUD Example, hear for Angular 6 CRUD Operation With PHP/MySQLi we will give you demo and example for implement.
In this post, we will learn about Angular 6 CRUD operation using PHP & MySQL with an example.
Angular 6 PHP CRUD (Create, Read, Update, Delete) Operations
There are the Following The simple About Angular 6 PHP CRUD (Create, Read, Update, Delete) Operations Full Information With Example and source code.
As I will cover this Post with live Working example to develop Angular 6 CRUD Operations with PHP and MySQL, so the some major files and Directory structures for this example is following below.
- Angular 6 OverView
- Angular 6 Project Structure
- Angular 6 Routing
- Spring Boot
- Service in Angular 6
- Creating Components
- Testing Angular 6
Angular 6 Example
Angular 6 crud example php Latest My Previous Post With Source code Read more…..
- Angular 6 Folder Project Structure
- Angular 6 CLI Installation
- Angular 6 Module File
- Angular 6 Components
- Angular 6 Services
- Angular 6 Routing
- Angular 6 CSS
- Angular 6 Class Binding
- Angular 6 Animation
- Angular 6 Templating
- Angular 6 HTTP Client
Angular 6 Project Structure
index.php
This is where I will make a simple HTML form and PHP server side source code for our web application. To make the forms simply all souce code copy and write it into your any text editor Like Notepad++, then save file it as index.php.
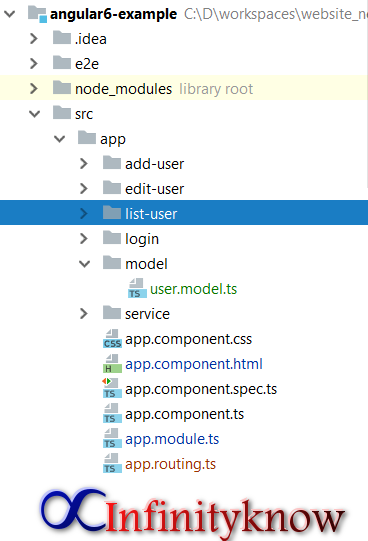
simple crud operation in Angular 6
Angular 6 Routing – Angular 6 crud example mvc
Angular 6 crud example mvc – app.routing.ts
[php]
import { RouterModule, Routes } from ‘@angular/router’;
import {LoginComponent} from “./login/login.component”;
import {AddStaffComponent} from “./add-staff/add-staff.component”;
import {ListStaffComponent} from “./list-staff/list-staff.component”;
import {EditStaffComponent} from “./edit-staff/edit-staff.component”;
const routes: Routes = [
{ path: ‘login’, component: LoginComponent },
{ path: ‘add-staff’, component: AddStaffComponent },
{ path: ‘list-staff’, component: ListStaffComponent },
{ path: ‘edit-staff’, component: EditStaffComponent },
{path : ”, component : LoginComponent}
];
export const routing = RouterModule.forRoot(routes);
[/php]
app.module.ts
[php]
import { BrowserModule } from ‘@angular/platform-browser’;
import { NgModule } from ‘@angular/core’;
import { AppComponent } from ‘./app.component’;
import { LoginComponent } from ‘./login/login.component’;
import {routing} from “./app.routing”;
import {AuthenticationService} from “./service/auth.service”;
import {ReactiveFormsModule} from “@angular/forms”;
import {HttpClientModule} from “@angular/common/http”;
import { AddStaffComponent } from ‘./add-staff/add-staff.component’;
import { EditStaffComponent } from ‘./edit-staff/edit-staff.component’;
import {ListStaffComponent} from “./list-staff/list-staff.component”;
import {StaffService} from “./service/staff.service”;
@NgModule({
declarations: [
AppComponent,
LoginComponent,
ListStaffComponent,
AddStaffComponent,
EditStaffComponent
],
imports: [
BrowserModule,
routing,
ReactiveFormsModule,
HttpClientModule
],
providers: [AuthenticationService, StaffService],
bootstrap: [AppComponent]
})
export class AppModule { }
[/php]
Service in Angular 6 Application
StaffService API Information Angular 6 CRUD
[php]
let fakeStaffs = [{id: 1, staffName: ‘krunal’, staffLname: ‘Ray’, email: ‘[email protected]’},
{id: 1, staffName: ‘jaydeep’, staffLname: ‘Jac’, email: ‘[email protected]’},
{id: 1, staffName: ‘ankit’, staffLname: ‘Pan’, email: ‘[email protected]’},
{id: 1, staffName: ‘vivek’, staffLname: ‘pb’, email: ‘[email protected]’},
];
return Observable.of(fakeStaffs).delay(5000);
[/php]
staff.service.ts
[php]
import { Injectable } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
import {Staff} from “../model/staff.model”;
@Injectable()
export class StaffService {
constructor(private http: HttpClient) { }
baseUrl: string = ‘http://localhost:8080/staff-portal/staffs’;
getStaffs() {
return this.http.get(this.baseUrl);
}
getStaffById(id: number) {
return this.http.get(this.baseUrl + ‘/’ + id);
}
createStaff(staff: Staff) {
return this.http.post(this.baseUrl, staff);
}
updateStaff(staff: Staff) {
return this.http.put(this.baseUrl + ‘/’ + staff.id, staff);
}
deleteStaff(id: number) {
return this.http.delete(this.baseUrl + ‘/’ + id);
}
}
[/php]
Creating Components in Angular 6
login.component.html
[php]
Login
[/php]
login.component.ts
[php]
import { Component, OnInit } from ‘@angular/core’;
import {FormBuilder, FormGroup, Validators} from “@angular/forms”;
import {Router} from “@angular/router”;
import {first} from “rxjs/operators”;
import {AuthenticationService} from “../service/auth.service”;
@Component({
selector: ‘app-login’,
templateUrl: ‘./login.component.html’,
styleUrls: [‘./login.component.css’]
})
export class LoginComponent implements OnInit {
loginForm: FormGroup;
submitted: boolean = false;
invalidLogin: boolean = false;
constructor(private formBuilder: FormBuilder, private router: Router, private authService: AuthenticationService) { }
onSubmit() {
this.submitted = true;
if (this.loginForm.invalid) {
return;
}
if(this.loginForm.controls.email.value == ‘[email protected]’ && this.loginForm.controls.password.value == ‘password’) {
this.router.navigate([‘list-staff’]);
}else {
this.invalidLogin = true;
}
}
ngOnInit() {
this.loginForm = this.formBuilder.group({
email: [”, Validators.required],
password: [”, Validators.required]
});
}
}
[/php]
Angular 6 PHP CRUD Operations – List
list-staff.component.html
[php]
Staff Details
Id | StaffName | LastName | Action | |
---|---|---|---|---|
[/php]
list-staff.component.ts
[php]
import { Component, OnInit } from ‘@angular/core’;
import {Router} from “@angular/router”;
import {StaffService} from “../service/staff.service”;
import {Staff} from “../model/staff.model”;
@Component({
selector: ‘app-list-staff’,
templateUrl: ‘./list-staff.component.html’,
styleUrls: [‘./list-staff.component.css’]
})
export class ListStaffComponent implements OnInit {
staffs: Staff[];
constructor(private router: Router, private staffService: StaffService) { }
ngOnInit() {
this.staffService.getStaffs()
.subscribe( data => {
this.staffs = data;
});
}
deleteStaff(staff: Staff): void {
this.staffService.deleteStaff(staff.id)
.subscribe( data => {
this.staffs = this.staffs.filter(u => u !== staff);
})
};
editStaff(staff: Staff): void {
localStorage.removeItem(“editStaffId”);
localStorage.setItem(“editStaffId”, staff.id.toString());
this.router.navigate([‘edit-staff’]);
};
addStaff(): void {
this.router.navigate([‘add-staff’]);
};
}
[/php]
Angular 6 PHP CRUD Operations – Add Form
add-staff.component.html
[php]
[/php]
add-staff.component.ts
[php]
import { Component, OnInit } from ‘@angular/core’;
import {FormBuilder, FormGroup, Validators} from “@angular/forms”;
import {StaffService} from “../service/staff.service”;
import {first} from “rxjs/operators”;
import {Router} from “@angular/router”;
@Component({
selector: ‘app-add-staff’,
templateUrl: ‘./add-staff.component.html’,
styleUrls: [‘./add-staff.component.css’]
})
export class AddStaffComponent implements OnInit {
constructor(private formBuilder: FormBuilder,private router: Router, private staffService: StaffService) { }
addForm: FormGroup;
ngOnInit() {
this.addForm = this.formBuilder.group({
id: [],
email: [”, Validators.required],
staffName: [”, Validators.required],
staffLname: [”, Validators.required]
});
}
onSubmit() {
this.staffService.createStaff(this.addForm.value)
.subscribe( data => {
this.router.navigate([‘list-staff’]);
});
}
}
[/php]
Angular 6 PHP CRUD Operations – Edit Form
edit-staff.component.html
[php]
Edit Staff
[/php]
edit-staff.component.ts
[php]
import { Component, OnInit } from ‘@angular/core’;
import {StaffService} from “../service/staff.service”;
import {Router} from “@angular/router”;
import {Staff} from “../model/staff.model”;
import {FormBuilder, FormGroup, Validators} from “@angular/forms”;
import {first} from “rxjs/operators”;
@Component({
selector: ‘app-edit-staff’,
templateUrl: ‘./edit-staff.component.html’,
styleUrls: [‘./edit-staff.component.css’]
})
export class EditStaffComponent implements OnInit {
staff: Staff;
editForm: FormGroup;
constructor(private formBuilder: FormBuilder,private router: Router, private staffService: StaffService) { }
ngOnInit() {
let staffId = localStorage.getItem(“editStaffId”);
if(!staffId) {
alert(“Invalid action.”)
this.router.navigate([‘list-staff’]);
return;
}
this.editForm = this.formBuilder.group({
id: [],
email: [”, Validators.required],
staffName: [”, Validators.required],
staffLname: [”, Validators.required]
});
this.staffService.getStaffById(+staffId)
.subscribe( data => {
this.editForm.setValue(data);
});
}
onSubmit() {
this.staffService.updateStaff(this.editForm.value)
.pipe(first())
.subscribe(
data => {
this.router.navigate([‘list-staff’]);
},
error => {
alert(error);
});
}
}
[/php]
Angular 6 php framework Examples
- Angular 6 applications – Insert, Fetch , Edit – update , Delete Operations
- Angular 6 CRUD
- Angular 6 and ASP.NET Core 2.0 Web API
- Angular 6 features
- Angular 6 Form Validation
- Angular 6 – User Registration and Login
- Angularjs 6 User Registration and Login Authentication
- Angular 6 CLI Installation Tutorial With Example
- Angular 6 Toast Message Notifications From Scratch
Read :
Summary
You can also read about AngularJS, ASP.NET, VueJs, PHP.
I hope you get an idea about Angular 6 php framework – CRUD Operations.
I would like to have feedback on my Infinityknow.com blog.
Your valuable feedback, question, or comments about this article are always welcome.
If you enjoyed and liked this post, don’t forget to share.